Xano MCP Server
Enables interaction with the Xano API through a Model Context Protocol (MCP) interface, providing secure and type-safe management of Xano database operations.
SarimSiddd
Tools
get_workspaces
Get all available workspaces
create_table
Create a new table in a workspace
get_table_content
Get content from a table
add_table_content
Add content to a table
update_table_content
Update content in a table
get_all_tables
Get all tables in a workspace
README
Xano MCP Server
A Model Context Protocol (MCP) server implementation for interacting with the Xano API. This server provides tools and resources for managing Xano database operations through the MCP interface.
Features
- Secure authentication with Xano API
- Type-safe API interactions using TypeScript
- Environment-based configuration
- MCP-compliant interface
- Workspace management tools
- Table content operations (create, read, update)
- Improved error handling with detailed messages
Installation
# Clone the repository
git clone [your-repo-url]
cd xano_mcp
# Install dependencies
npm install
Configuration
- Copy the example environment file:
cp .env.example .env
- Update the
.env
file with your Xano credentials:
XANO_API_KEY=your_api_key_here
XANO_API_URL=your_xano_api_url
NODE_ENV=development
API_TIMEOUT=10000
Development
# Build the project
npm run build
# Run in development mode
npm run dev
# Start the server
npm start
Project Structure
xano_mcp/
├── src/
│ ├── api/
│ │ └── xano/
│ │ ├── client/ # API client implementation
│ │ ├── models/ # Data models and types
│ │ ├── services/ # API service implementations
│ │ └── utils/ # Utility functions
│ ├── mcp/
│ │ ├── server/ # MCP server implementation
│ │ ├── tools/ # MCP tool implementations
│ │ └── types/ # Tool-specific types
│ ├── config.ts # Configuration management
│ └── index.ts # Main entry point
├── .env # Environment variables (not in git)
├── .env.example # Example environment variables
└── tsconfig.json # TypeScript configuration
Available MCP Tools
Workspace Tools
get_workspaces
: List all available workspaces
Table Tools
create_table
: Create a new table in a workspaceget_table_content
: Get content from a table with pagination supportadd_table_content
: Add new content to a tableupdate_table_content
: Update existing content in a tableget_all_tables
: List all tables in a workspace with detailed information
Usage Examples
Working with Workspaces
// List available workspaces
const result = await mcp.use_tool("get_workspaces", {});
console.log('Workspaces:', result);
Managing Tables
// Create a new table
const createResult = await mcp.use_tool("create_table", {
workspaceId: 123,
name: "MyTable"
});
// Add content to a table
const addResult = await mcp.use_tool("add_table_content", {
workspaceId: 123,
tableId: 456,
content: {
created_at: "2024-01-22T17:07:00.000Z"
}
});
// Get table content with pagination
const getResult = await mcp.use_tool("get_table_content", {
workspaceId: 123,
tableId: 456,
pagination: {
page: 1,
items: 50
}
});
// Update table content
const updateResult = await mcp.use_tool("update_table_content", {
workspaceId: 123,
tableId: 456,
contentId: "789",
content: {
created_at: "2024-01-22T17:07:00.000Z"
}
});
// List all tables in a workspace
const tables = await mcp.use_tool("get_all_tables", {
workspaceId: 123
});
console.log('Tables:', tables);
// Returns an array of tables with their details:
// [
// {
// id: number,
// name: string,
// description: string,
// created_at: string,
// updated_at: string,
// guid: string,
// auth: boolean,
// tag: string[],
// workspaceId: number
// },
// ...
// ]
Environment Variables
Variable | Description | Required | Default |
---|---|---|---|
XANO_API_KEY | Your Xano API authentication key | Yes | - |
XANO_API_URL | Xano API endpoint URL | Yes | - |
NODE_ENV | Environment (development/production) | No | development |
API_TIMEOUT | API request timeout in milliseconds | No | 10000 |
Error Handling
The server provides detailed error messages for:
- Invalid parameters
- Authentication failures
- API request failures
- Content validation errors
- Unknown tool requests
Security
- Environment variables are used for sensitive configuration
- TruffleHog configuration is included to prevent secret leaks
- API keys and sensitive data are never committed to the repository
Contributing
- Create a feature branch
- Make your changes
- Submit a pull request
License
ISC
Recommended Servers
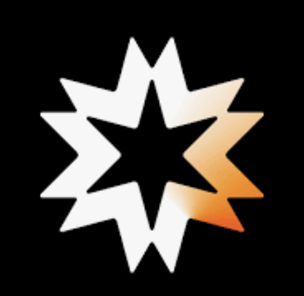
E2B
Using MCP to run code via e2b.
AIO-MCP Server
🚀 All-in-one MCP server with AI search, RAG, and multi-service integrations (GitLab/Jira/Confluence/YouTube) for AI-enhanced development workflows. Folk from
React MCP
react-mcp integrates with Claude Desktop, enabling the creation and modification of React apps based on user prompts
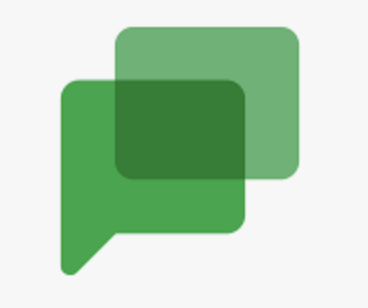
Any OpenAI Compatible API Integrations
Integrate Claude with Any OpenAI SDK Compatible Chat Completion API - OpenAI, Perplexity, Groq, xAI, PyroPrompts and more.
Exa MCP
A Model Context Protocol server that enables AI assistants like Claude to perform real-time web searches using the Exa AI Search API in a safe and controlled manner.
MySQL Server
Allows AI assistants to list tables, read data, and execute SQL queries through a controlled interface, making database exploration and analysis safer and more structured.
Browser Use (used by Deploya.dev)
AI-driven browser automation server that implements the Model Context Protocol to enable natural language control of web browsers for tasks like navigation, form filling, and visual interaction.
Aindreyway Codex Keeper
Serves as a guardian of development knowledge, providing AI assistants with curated access to latest documentation and best practices.

OpenRouter MCP Server
Provides integration with OpenRouter.ai, allowing access to various AI models through a unified interface.
Supabase MCP Server (used by Deploya.dev)
Enables Cursor and Windsurf to safely interact with Supabase databases by providing tools for database management, SQL query execution, and Supabase Management API access with built-in safety controls.