
Rand
Provides random number generation utilities, including a secure UUID generator powered by Node's crypto module.
turlockmike
Tools
roll_dice
Roll a set of dice using standard dice notation (e.g., "2d6" for two six-sided dice, "3d6+5" for three six-sided dice plus 5)
generate_uuid
Generate a random UUID v4
generate_random_number
Generate a random number within a specified range
generate_gaussian
Generate a random number following a Gaussian (normal) distribution between 0 and 1
generate_string
Generate a random string with specified length and character set
draw_cards
Draw cards from a standard deck of playing cards
generate_password
Generate a strong password with a mix of character types. WARNING: While this password is generated locally on your machine, it is recommended to use a dedicated password manager for generating and storing passwords securely.
README
MCP Rand
A Model Context Protocol (MCP) server providing various random generation utilities, including UUID, numbers, strings, passwords, Gaussian distribution, dice rolling, and card drawing.
<a href="https://glama.ai/mcp/servers/ccd6b0hni8"><img width="380" height="200" src="https://glama.ai/mcp/servers/ccd6b0hni8/badge" alt="Rand MCP server" /></a>
Installation
npm install mcp-rand
Or install globally:
npm install -g mcp-rand
Features
UUID Generator
- Generates RFC 4122 version 4 UUIDs
- Uses Node's native crypto module for secure random generation
- No parameters required
Random Number Generator
- Generates random numbers within a specified range
- Configurable minimum and maximum values (inclusive)
- Defaults to range 0-100 if no parameters provided
Gaussian Random Generator
- Generates random numbers following a Gaussian (normal) distribution
- Normalized to range 0-1
- No parameters required
Random String Generator
- Generates random strings with configurable length and character sets
- Supports multiple character sets:
- alphanumeric (default): A-Z, a-z, 0-9
- numeric: 0-9
- lowercase: a-z
- uppercase: A-Z
- special: !@#$%^&*()_+-=[]{};'"\|,.<>/?
- Configurable string length (defaults to 10)
Password Generator
- Generates strong passwords with a mix of character types
- Ensures at least one character from each type (uppercase, lowercase, numbers, special)
- Configurable length (minimum 8, default 16)
- WARNING: While passwords are generated locally, it's recommended to use a dedicated password manager
Dice Roller
- Roll multiple dice using standard dice notation
- Supports notation like "2d6" (two six-sided dice), "1d20" (one twenty-sided die)
- Returns individual rolls and total for each set of dice
- Can roll multiple different dice sets at once (e.g., "2d6", "1d20", "4d4")
Card Drawer
- Draw cards from a standard 52-card deck
- Maintains deck state between draws using base64 encoding
- Returns drawn cards and remaining deck state
- Supports drawing any number of cards up to the deck size
- Properly shuffles available cards before each draw
Usage
As a CLI Tool
npx mcp-rand
Integration with MCP Clients
Add to your MCP client configuration:
{
"mcpServers": {
"mcp-rand": {
"command": "node",
"args": ["path/to/mcp-rand/build/index.js"],
"disabled": false,
"alwaysAllow": []
}
}
}
Example Usage
// Generate UUID
const uuid = await client.callTool('generate_uuid', {});
console.log(uuid); // e.g., "550e8400-e29b-41d4-a716-446655440000"
// Generate random number
const number = await client.callTool('generate_random_number', {
min: 1,
max: 100
});
console.log(number); // e.g., 42
// Generate Gaussian random number
const gaussian = await client.callTool('generate_gaussian', {});
console.log(gaussian); // e.g., 0.6827
// Generate random string
const string = await client.callTool('generate_string', {
length: 15,
charset: 'alphanumeric'
});
console.log(string); // e.g., "aB9cD8eF7gH6iJ5"
// Generate password
const password = await client.callTool('generate_password', {
length: 20
});
console.log(password); // e.g., "aB9#cD8$eF7@gH6*iJ5"
// Roll dice
const rolls = await client.callTool('roll_dice', {
dice: ['2d6', '1d20', '4d4']
});
console.log(rolls);
/* Output example:
[
{
"dice": "2d6",
"rolls": [3, 1],
"total": 4
},
{
"dice": "1d20",
"rolls": [4],
"total": 4
},
{
"dice": "4d4",
"rolls": [2, 3, 2, 3],
"total": 10
}
]
*/
// Draw cards
const draw1 = await client.callTool('draw_cards', {
count: 5
});
console.log(draw1);
/* Output example:
{
"drawnCards": [
{ "suit": "hearts", "value": "A" },
{ "suit": "diamonds", "value": "7" },
{ "suit": "clubs", "value": "K" },
{ "suit": "spades", "value": "2" },
{ "suit": "hearts", "value": "10" }
],
"remainingCount": 47,
"deckState": "t//+///bDw=="
}
*/
// Draw more cards using previous deck state
const draw2 = await client.callTool('draw_cards', {
count: 3,
deckState: draw1.deckState
});
console.log(draw2);
/* Output example:
{
"drawnCards": [
{ "suit": "diamonds", "value": "Q" },
{ "suit": "clubs", "value": "5" },
{ "suit": "spades", "value": "J" }
],
"remainingCount": 44,
"deckState": "l//+//zbDw=="
}
*/
Contributing
Please see CONTRIBUTING.md for development setup and guidelines.
License
ISC
Recommended Servers
AIO-MCP Server
🚀 All-in-one MCP server with AI search, RAG, and multi-service integrations (GitLab/Jira/Confluence/YouTube) for AI-enhanced development workflows. Folk from
Persistent Knowledge Graph
An implementation of persistent memory for Claude using a local knowledge graph, allowing the AI to remember information about users across conversations with customizable storage location.
React MCP
react-mcp integrates with Claude Desktop, enabling the creation and modification of React apps based on user prompts
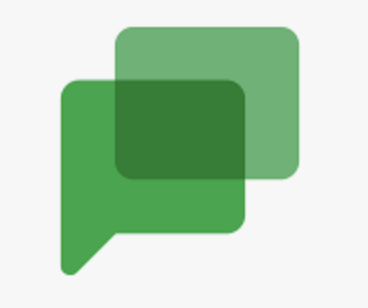
Any OpenAI Compatible API Integrations
Integrate Claude with Any OpenAI SDK Compatible Chat Completion API - OpenAI, Perplexity, Groq, xAI, PyroPrompts and more.
Exa MCP
A Model Context Protocol server that enables AI assistants like Claude to perform real-time web searches using the Exa AI Search API in a safe and controlled manner.
AI 图像生成服务
可用于cursor 集成 mcp server
Web Research Server
A Model Context Protocol server that enables Claude to perform web research by integrating Google search, extracting webpage content, and capturing screenshots.
MySQL Server
Allows AI assistants to list tables, read data, and execute SQL queries through a controlled interface, making database exploration and analysis safer and more structured.
Browser Use (used by Deploya.dev)
AI-driven browser automation server that implements the Model Context Protocol to enable natural language control of web browsers for tasks like navigation, form filling, and visual interaction.
Aindreyway Codex Keeper
Serves as a guardian of development knowledge, providing AI assistants with curated access to latest documentation and best practices.